In order to get the PUMA robots working I need to be able to control their brushed DC motors, but have not been satisfied with any of the off the shelf motor drivers. Servo drives for brushed DC motors seem surprisingly hard to come by. And those that exist are either more expensive than I am looking to spend for this project, are lacking features (gravity compensation/compliancy), or are not rated for the current of the PUMA motors. So I have taken to making my own, currently based on the Teensy 4.0. More on the drives in a future post. But I needed a way to count the quadrature encoder pulses from the servos.
Now I probably could have used the Teensy’s built-in encoder counting, but I feel more comfortable off loading all the encoder functions to a dedicated IC that could also be used with other microcontrollers as well.
What I ended up with is the LSI LS7366R quadrature counter. A 32 bit, 5 volt, SPI based chip. It is fairly straightforward to implement, and I’ve written a simple Arduino based library to interface with it, LS7366R_Lib.
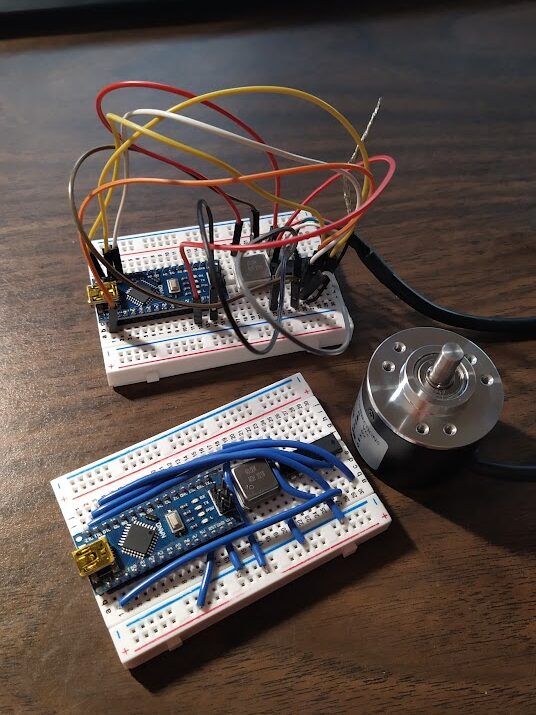
The IC has a count frequency of 40Mhz when operating at 5v or 20Mhz when operating at 3v, requiring a corresponding clock signal, for which I use a crystal oscillator.
The full code for using this IC can be found open-source on Github.
The code below shows how to setup and use the library for basic quadrature counting on using an Arduino or compatible board, such as a Teensy.
#include "LS7366R_Lib.h"
LS7366R_Lib encoder(9);
uint32_t testInt = 1000; // Value to start the counter at.
void setup() {
Serial.begin(115200);
encoder.begin();
encoder.set_MDR0(x4, free_run, disable_index, async, filter_1);
encoder.set_MDR1(byte_4, count_ena, nop, nop, nop, nop);
encoder.clear_CNTR();
encoder.set_DTR(testInt);
encoder.load_CNTR(); // Sets the counter to start at 1,000.
}
void loop() {
encoder.load_OTR();
Serial.println(encoder.get_OTR());
delay(1000);
}
The SPI interface of this chip allows for very fast communication between the microcontroller and the encoder IC, crucial when running high speed control loops.
This library implements all of the functions that the LS7366R is capable of including…
- Counting from 1 to 4 quadrature counts per quadrature cycle or non-quadrature mode using the A phase input as a count signal and B as a direction.
- Optional index pulse for resetting or transferring values between it’s internal registers.
- Programable 1 to 4 byte count resister width.
A full list of the functions and how to use them are found on the github page or on the product datasheet here.